Improving the Efficiency of My Solar Panel Monitoring Plugin: Optimising PHP, MySQL, and Caching
As a part of my ongoing efforts to optimise and enhance the functionality of my solar panel monitoring plugin, I’ve recently made some significant improvements that have boosted its performance and security. If you’re interested in solar generation data or just want to know how to improve the efficiency of a WordPress plugin, this post will walk you through the key changes I’ve made—streamlining PHP and MySQL, particularly through caching, as well as enhancing security in the process.
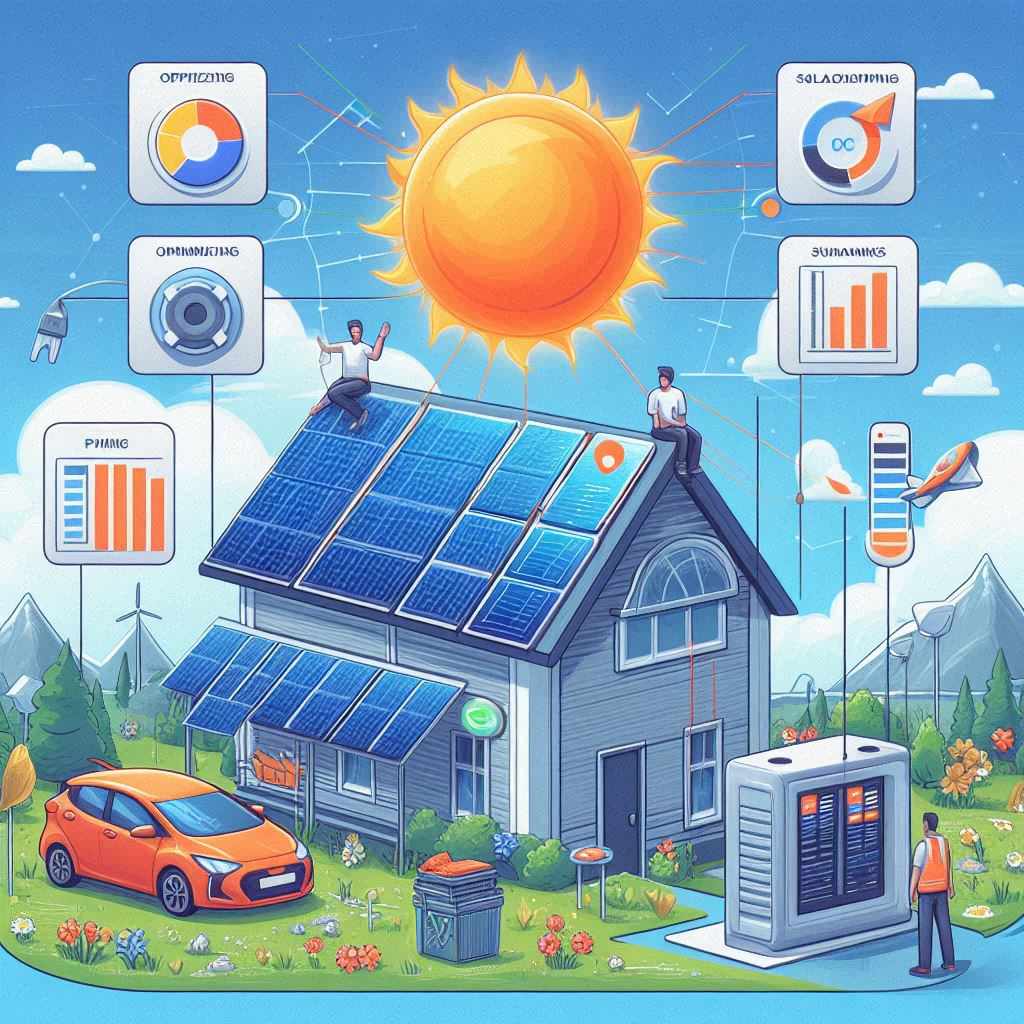
Why Efficiency Matters
When it comes to tracking solar panel performance, the accuracy and speed of data retrieval are crucial. My plugin interfaces with MySQL databases to pull solar generation stats in real time. As the years roll by, and the database increases in size daily, I noticed that the plugin’s performance could be optimised further. There was an increasing delay in fetching and displaying the data as the amount of data to crunch grew. The key to improving speed was reducing the number of database calls and optimising the way information is retrieved and cached.
Streamlining PHP and MySQL
The heart of my plugin lies in the PHP code and MySQL database queries. Initially, the plugin executed a number of individual MySQL queries each time the user accessed the solar generation data. With growing traffic, this created a bottleneck, leading to slower load times and an increase in server load.
1. Refactoring PHP Code: The first step I took was refactoring the PHP code to reduce redundant queries. Instead of making multiple calls to the database for related data, I consolidated them into fewer, more efficient queries. This helped decrease server load and reduced the amount of processing required on each request.
2. Optimising MySQL Queries: Next, I focused on optimising the MySQL queries. Many of the queries could be more efficient with better indexing and less reliance on complex queries. By streamlining the queries and ensuring that only the necessary data was being pulled, I reduced the load on the database and made the retrieval process faster.
Leveraging Transients for Caching
One of the most effective ways I improved efficiency was by implementing caching. Caching is the process of storing frequently accessed data temporarily so it doesn’t need to be re-fetched every time it’s requested. In WordPress, transients provide an easy way to store cached data with an expiration time.
Here’s how transients helped:
- Database Query Caching: I started using transients to cache the results of MySQL queries. For example, when pulling solar generation data from the database, I now store the results in a transient for a set period (e.g., 3 hours). During that time, the plugin retrieves the cached data instead of executing a new query. This dramatically reduces the number of database queries and speeds up the process for users. Some data, such as the information about yesterday’s solar yield, only really needs updating once a day, so the cache on those can be even longer. Rather than querying the database every minute to get this information it’s better to query it a couple of times a day and store it in a cache.
- Automatic Expiration: Transients automatically expire after a specified time, which means the data that changes regularly can remain relatively fresh while still avoiding unnecessary database calls. This balances performance with the need for accuracy.
Code Example: Here’s an example of how I implemented the transient caching for one of the key queries:
// Ensure the date format is in 'YYYY-MM-DD'
$yesterdays_formatted = date('Y-m-d', ($yesterdays_date)));
// Cache key for yesterday's query
$yesterdays_cache_key = 'yesterdays_query_' . $yesterdays_formatted;
// Check if the result is already cached
$yesterdaysquery = get_transient($yesterdays_cache_key);
// If cache is not found, run the query and cache the result
if ($yesterdaysquery === false) {
// Prepared statement for security
$yesterdaysquery = $wpdb->get_results(
$wpdb->prepare(
"SELECT * FROM $table_name WHERE date = %s LIMIT 1",
$yesterdays_formatted
)
);
// Store the result in cache for 4 hours (14,400 seconds)
set_transient($yesterdays_cache_key, $yesterdaysquery, 14400);
}
By implementing this caching mechanism, I’ve reduced the number of queries executed each time someone visits the plugin’s page, improving speed and efficiency.
Enhancing Security
While performance was a top priority, security is always a crucial consideration. By streamlining the PHP code and MySQL queries, I also took steps to make sure the plugin remained secure:
- SQL Injection Prevention: One of the key improvements was making sure all MySQL queries are properly sanitised to prevent SQL injection attacks. I used prepared statements for dynamic queries, which ensures that user inputs cannot interfere with the structure of the query itself.
- User Input Validation: Additionally, I made sure to validate any user inputs before executing queries. This is critical in avoiding potential vulnerabilities.
Results: Faster, More Secure, and More Efficient
By optimising the PHP code, MySQL queries, and leveraging caching with transients, the performance of the solar panel monitoring plugin has greatly improved. Here are the key results:
- Faster Load Times: Reduced the number of database queries and sped up the page load time.
- Lower Server Load: Caching queries reduces the pressure on the server, making it more scalable as traffic grows.
- Improved Security: Proper input validation and sanitisation ensure the plugin remains secure for users.
These changes have made the plugin more efficient and secure, and I’m excited to continue enhancing it. Whether you’re running a similar plugin or just want to learn more about optimising WordPress plugins in general, I hope these insights help you in your own development efforts.
Conclusion
If you’re looking to improve the efficiency of your WordPress plugin, whether it’s related to solar panel monitoring or any other data-driven task, consider refactoring your PHP code, optimising your MySQL queries, and implementing caching with transients. Not only will it speed up the plugin, but it will also reduce server load, improve user experience, and strengthen security.
Check out my plugin and monitor the performance improvements on the Solar Generation page. Happy coding!
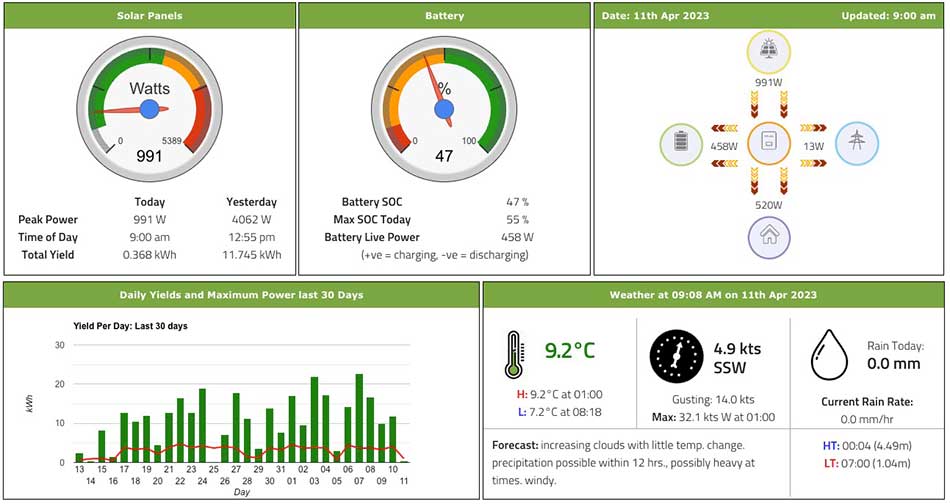